
30 Python Programming Questions on Conditional Statements
Q 1. Check if a number is positive, negative, or zero.
Input: num = 7
Expected Output:
Positive
Q 2. Determine if a year is a leap year.
Input: year = 2024
Expected Output:
Leap Year
Q 3. Check if a number is even or odd.
Input: num = 10
Expected Output:
Even
Q 4. Determine if a number is within a specific range.
Input: num = 25
Expected Output:
Out of range
Q 5. Compare two numbers and print the greater one.
Input: a = 5, b = 8
Expected Output:
8
Q 6. Check if a person is eligible to vote (age >= 18).
Input: age = 16
Expected Output:
Not eligible to vote
Q 7. Check if a number is divisible by both 3 and 5.
Input: num = 15
Expected Output:
Divisible by both 3 and 5
Q 8. Determine if a number is positive or non-positive.
Input: num = -3
Expected Output:
Non-positive
Q 9. Check if a string length is greater than 10.
Input: string = "Hello World"
Expected Output:
Length is greater than 10
Q 10. Check if a character is a vowel.
Input: char = 'e'
Expected Output:
Vowel
Q 11. Determine if a number is greater than 100.
Input: num = 150
Expected Output:
Greater than 100
Q 12. Check if a given number is between 1 and 10 inclusive.
Input: num = 7
Expected Output:
Within range
Q 13. Print the largest of three numbers.
Input: a = 10, b = 20, c = 15
Expected Output:
20
Q 14. Check if a number is divisible by 7.
Input: num = 14
Expected Output:
Divisible by 7
Q 15. Check if a person is an adult or a minor (age >= 18).
Input: age = 20
Expected Output:
Adult
Q 16. Determine the grade based on the score.
Input: score = 85
Expected Output:
Grade B
Q 17. Check if a number is a multiple of 10.
Input: num = 50
Expected Output:
Multiple of 10
Q 18. Determine if a number is positive and less than 100.
Input: num = 45
Expected Output:
Positive and less than 100
Q 19. Print the appropriate message for the day of the week.
Input: day = 'Wednesday'
Expected Output:
Midweek
Q 20. Check if a number is positive or negative, and also if it’s even or odd.
Input: num = -8
Expected Output:
Negative Even
Q 21. Determine if a person is eligible for senior citizen benefits (age >= 65).
Input: age = 70
Expected Output:
Eligible for senior citizen benefits
Q 22. Check if a number is within 1 to 100.
Input: num = 85
Expected Output:
Number is within 1 to 100
Q 23. Print the smallest of three numbers.
Input: a = 12, b = 8, c = 15
Expected Output:
8
Q 24. Check if a number is positive and divisible by 3.
Input: num = 9
Expected Output:
Positive and divisible by 3
Q 25. Determine if a number is greater than or equal to 50 but less than 100.
Input: num = 75
Expected Output:
Number is in the range 50 to 99
Q 26. Check if a number is a multiple of both 2 and 5.
Input: num = 20
Expected Output:
Multiple of both 2 and 5
Q 27. Determine if a given month number corresponds to a winter month (December, January, February).
Input: month = 1
Expected Output:
Winter
Q 28. Check if a given number is a multiple of 6.
Input: num = 18
Expected Output:
Multiple of 6
Q 29. Determine if a number is either less than 10 or greater than 100.
Input: num = 5
Expected Output:
Number is less than 10 or greater than 100
Q 30. Print the message based on the grade obtained.
Input: grade = 'B'
Expected Output:
Good
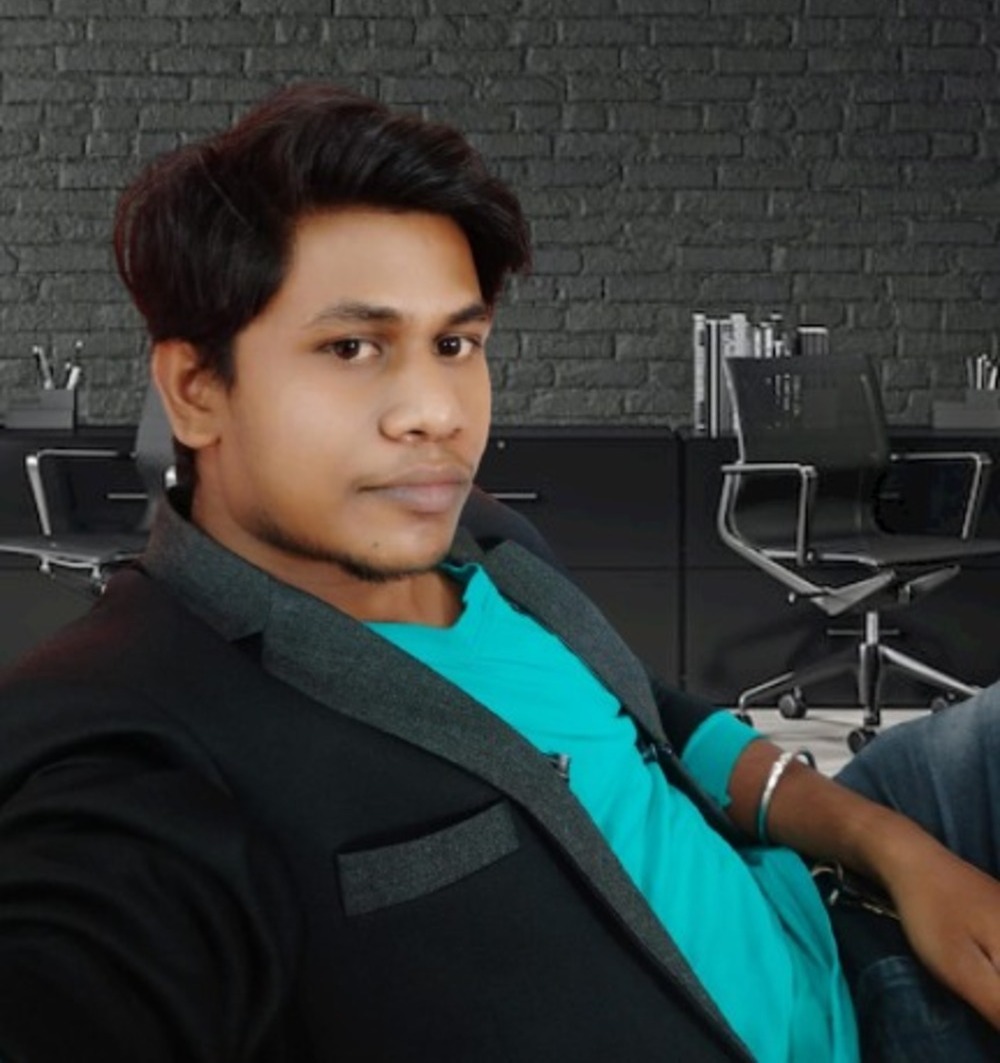
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!